how to add slider to widget anywhere:
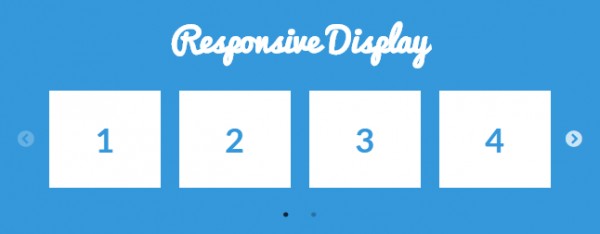
for this you should follow 4 simple steps:
1. Create a new widget by widget anywhere plugin called for example "Slider"
2. Download "Slick" from https://github.com/kenwheeler/slick then upload/extract it to your server
3. Add the following code to the head of every page from Admin> Layout
<link rel="stylesheet" type="text/css" href="http://yourdomain/path/to/slick-master/slick/slick.css"/>
<link rel="stylesheet" type="text/css" href="http://yourdomain/path/to/slick-master/slick/slick-theme.css"/>
4. In the widget "Slider" you have created add your slides like this:
<div class="your-class">
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
<div>Slide 4</div>
<div>Slide 5</div>
</div>
<script type="text/javascript" src="//code.jquery.com/jquery-1.11.0.min.js"></script>
<script type="text/javascript" src="//code.jquery.com/jquery-migrate-1.2.1.min.js"></script>
<script type="text/javascript" src="http://yourdomain/path/to/slick-master/slick/slick.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('.your-class').slick({
dots: true,
infinite: false,
speed: 300,
slidesToShow: 4,
slidesToScroll: 4,
responsive: [
{
breakpoint: 1024,
settings: {
slidesToShow: 3,
slidesToScroll: 3,
infinite: true,
dots: true
}
},
{
breakpoint: 600,
settings: {
slidesToShow: 2,
slidesToScroll: 2
}
},
{
breakpoint: 480,
settings: {
slidesToShow: 1,
slidesToScroll: 1
}
}
// You can unslick at a given breakpoint now by adding
// settings: "unslick"
// instead of a settings object
]
});
});
</script>
Here I just created 5 slides, you could create more slides just by adding
<div> Slide 6</div>
to
<div class="your-class">
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
<div>Slide 4</div>
<div>Slide 5</div>
</div>
For more settings you could see here: http://kenwheeler.github.io/slick/
That's all.
You could add slides to every other widgets or pages in a same way.
And you could also add this feature to your website without downloading Slick:
Add
<link rel="stylesheet" type="text/css" href="//cdn.jsdelivr.net/jquery.slick/1.5.9/slick.css"/>
// Add the slick-theme.css if you want default styling
<link rel="stylesheet" type="text/css" href="//cdn.jsdelivr.net/jquery.slick/1.5.9/slick-theme.css"/>
to the head of every page.
and then add
<div class="your-class">
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
<div>Slide 4</div>
<div>Slide 5</div>
</div>
<script type="text/javascript" src="//cdn.jsdelivr.net/jquery.slick/1.5.9/slick.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('.your-class').slick({
dots: true,
infinite: false,
speed: 300,
slidesToShow: 4,
slidesToScroll: 4,
responsive: [
{
breakpoint: 1024,
settings: {
slidesToShow: 3,
slidesToScroll: 3,
infinite: true,
dots: true
}
},
{
breakpoint: 600,
settings: {
slidesToShow: 2,
slidesToScroll: 2
}
},
{
breakpoint: 480,
settings: {
slidesToShow: 1,
slidesToScroll: 1
}
} // You can unslick at a given breakpoint now by adding
// settings: "unslick"
// instead of a settings object
]
});
});
</script>
to the widget yo have created
I hope it will be useful.